In any programming language, you will find yourself needing and using functions as a primary building block for your software development. In Python, functions are equally important, and we will discuss them in detail today.
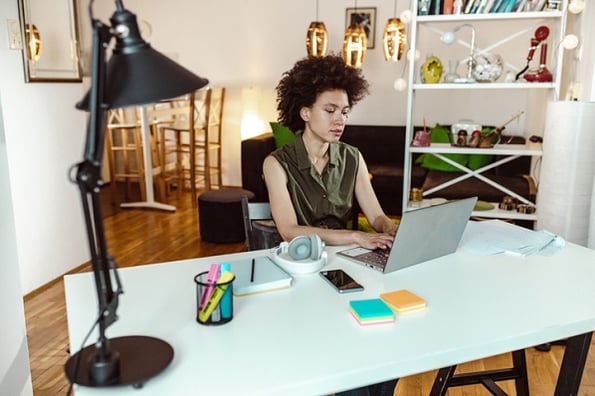
This post will cover the core concepts behind declaring, building, and calling a Python function. You will also learn how they work and discover the general syntax for them through some code examples showcasing actual use case scenarios.
Let's get started!
What is a function in Python?
A function is a block of code reused as needed throughout your program or software application. Well-written code should be modular and reusable, and functions support that goal. They provide a way to write cleaner, more efficient code, decrease repetition and improve overall performance.
Functions also further support object-oriented programming architecture, a coding style that supports modular code. But the question remains why use functions to accomplish these benefits? Moreover, how do they help with them?
Why do we use functions in Python?
Functions are commonly used blocks of code in programming for several reasons, some of which are named above. But the actual benefits are only seen when you understand what Python functions are fully capable of accomplishing.
Within functions, you can run many different expressions against many other arguments. You can perform loops, conditional expressions, run switch statements, and even construct or deconstruct collections such as Python lists.
But the real benefit is evident when you realize that you can reuse them often. When you combine these two points, you can see how functions can improve development.
Now that we've covered that, let's look at the syntax and declaration of Python functions.
How to Write Functions in Python
Writing Python functions is a simple task, and the complexity of the function is entirely dependent on the needs of the software source code and developer.
Let's start by looking at the syntax for defining a function.
The above pseudo-code demonstrates the general syntax for creating a function; you start with the keyword def, then the name you want to give your function. Then you declare any arguments the function may need to do what it needs.
Check out this next video to learn more about how Python functions are defined.
It is important to note that in Python, proper indentation is vital. If the indentation is incorrect, you will get an error when you run the code.
Finally, you can finish it with an optional return statement to send a value back. This example is straightforward to help explain the syntax and structure of a Python function. Now that you understand what they look like let's dive deeper into some simple examples of a few different functions.
Python Function Example
In this section, you will discover a few examples of Python functions to showcase how they work and what they can do for your development. With Python functions, you can define four types of arguments in your function.
Required arguments
Required arguments are exactly that, arguments that are required when you call the function. These arguments must all be satisfied and done so in the correct order.
The above function expects a value when it gets called, and it fails due to being called with no value passed into it. In this case, the error would indicate that the function expects one argument and zero were passed.
Keyword arguments
Keyword arguments are slightly different as they get passed into the function call as a variable declaration. Instead of simply passing in a variable or a value, you provide a value while assigning it to the defined arguments name.
This type of keyword can be advantageous in cases where you may not be certain in what order the arguments will get passed into the function call. Since the keyword arguments rely on the value assigned directly to them, their order is irrelevant.
Default arguments
Default arguments are helpful for optional arguments but provide a default value. They allow assigning default values to the argument in the function definition. But if the function gets called and a value is provided, it will overwrite the defined default with the one provided. Default arguments must be placed last in the argument list.
The code above would result in the string "Hello from the other side" printed to the console.
Variable-length arguments
Variable-length arguments are arguments that can accept multiple values stored as a tuple. These values can be accessed by the function from a single argument. Like default arguments, they must be defined last, which helps identify them. However, an asterisk is placed at the beginning of the argument name, signifying that it may have multiple values.
When the function runs, this code will result in the strings below being printed to the console.
This type of argument can help collect optional user information or allow users to add their information as they want. In that situation, you might not know how much information they will provide at a time.
One more thing to consider when working with functions is called scope. When declaring variables, you need to consider the scope from which you want the variable to be accessible. In Python, there are two basic scope levels — global and local. Global scope is the scope outside of a function and is available to anything in the program globally.
Local scope is the scope within a function that is only accessible within it; that is to say, it cannot be accessed by anything outside the function.
Moving Forward With Python Functions
This post has taught you everything you need to start working with python functions. But understanding the true power of functions is best found in working with them by putting them to use in your code. You can also learn about the Python Lambda function, a simple anonymous function that can be used in your functions. There is still a lot to learn, but you are now well on your way!