When it comes to programming, handling inputs efficiently is key for a user-friendly program. How inputs are managed can significantly impact the user experience and the overall success of a program.
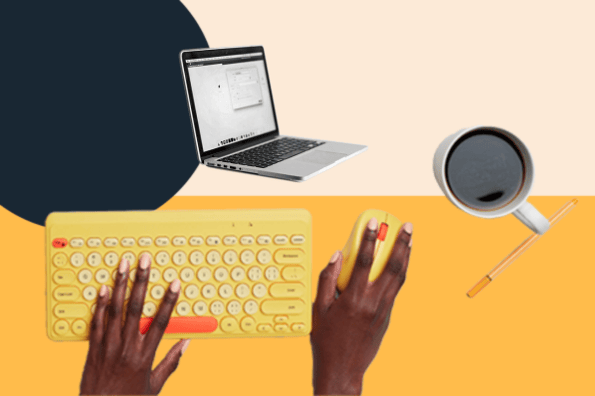
Python provides a wide range of tools for managing inputs effectively, from the built-in input() function to more advanced modules like argparse and regular expressions. However, for this blog, we will focus on the basics of handling user inputs in Python.
This post will dive into the world of Python's input() functions and explore the best practices for using them effectively. By the end of this post, you'll have a better understanding of how to handle inputs in your Python programs. So let's get started!
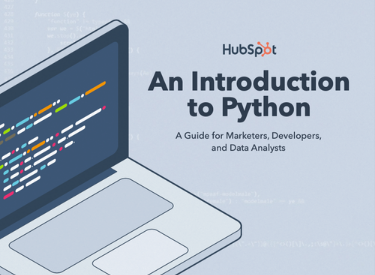
An Introduction to Python
A guide for marketers, developers, and data analysts. You'll learn about...
- What Python is.
- Programming Best Practices.
- Coding Standards.
- And More!
Download Free
All fields are required.
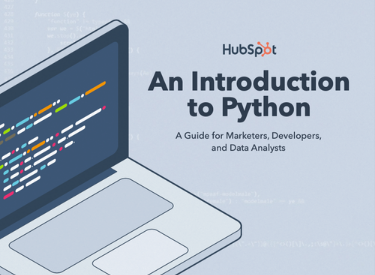
What is the input() function in Python?
The input() function is a built-in Python function that allows you to prompt the user for input and store it as a string. It's a simple and straightforward way to handle user inputs, making it a popular choice for many programmers.
How to use input() in Python?
The input() function reads a line of text from the user and returns it as a string. It takes an optional prompt argument, which is a string that will be displayed to the user before waiting for input.
When using the input() function, it's important to keep in mind that any data entered will be treated as a string by default. This means that any data entered must be passed through an appropriate conversion function to convert it into the desired type (e.g. int(), float(), etc.).
Here's an example of how you can use the input() function in your program:
In this example, the input() function prompts the user for their name and stores the input as a string in the variable name. The program then uses the variable to print a personalized greeting message.
When you call the input() function, Python waits for the user to enter a line of text and press the Enter key. It then reads the line and returns it as a string. If you don't provide a prompt argument, the input() function will simply wait for the user to enter text.
Handling Different Data Types With input()
As we discussed, by default, the input() function returns the user's input as a string. If you need to work with other data types, you can use type conversion functions like int(), float(), and bool() to convert the input string into the desired type.
In this example, the input() function prompts the user for their age and stores the input as a string. The program then uses the int() function to convert the string into an integer and checks if the user is old enough to enter.
Dealing with User Input Errors
Despite our best efforts, users will sometimes enter incorrect data or unexpected input. In such cases, it's important to handle these errors gracefully to prevent your program from crashing or providing incorrect output.
Using Try-Except Blocks
One of Python's most common ways to handle input errors is to use try-except blocks. A try-except block allows you to try a block of code and catch any exceptions that occur so that you can handle them appropriately.
Here's an example of how to use a try-except block to handle an input error:
In this example, we attempt to convert the user's input to an integer using the int() function. However, if the user enters a non-numeric value, a ValueError will be raised. We catch this exception using a try-except block and print a helpful error message to the user.
Input Validation
Another way to handle input errors is to validate the user's input before processing it. This can involve checking for specific data types, range limits, or other conditions that your program requires.
Here's an example of how to use input validation to check for a specific range of values:
In this example, we use a while loop to keep prompting the user for their age until a valid value between 0 and 120 is entered. We use input validation to ensure that the user's input meets our program's requirements, and we handle any errors that occur using a try-except block.
Providing Feedback to the User
When handling input errors, it's important to provide clear and helpful feedback to the user. This can include error messages, prompts for additional input, or other forms of guidance to help the user correct their input.
Here's an example of how to provide feedback to the user when handling input errors:
In this example, we provide feedback to the user depending on the validity of their input. If the user enters an age outside the specified range, we provide an error message and prompt them to enter a valid value. If the user enters a valid value, we print their age to the console.
Best Practices for Using input() Function
Here are some best practices to keep in mind when using the input() function:
- Always include a prompt argument to provide clear direction for the user.
- Use type conversion functions when necessary to convert input strings into other data types.
- Validate user input with conditional statements to ensure the data entered is valid and meets your expectations.
- Use try-except blocks to handle errors that might occur when processing user input.
- Avoid using the input() function for sensitive information like passwords, as the user's input will be displayed in plain text.
We explored the basics of Python's input() function and provided tips and tricks for handling user inputs effectively in your programs. By following best practices for handling input errors, using input validation, and providing clear feedback to the user, you can create user-friendly programs that provide a smooth and enjoyable experience for your users. With these techniques under your belt, you're now ready to master the art of Python input handling and take your programming skills to the next level.
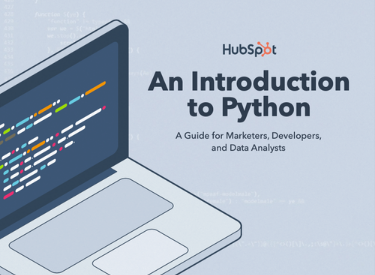
An Introduction to Python
A guide for marketers, developers, and data analysts. You'll learn about...
- What Python is.
- Programming Best Practices.
- Coding Standards.
- And More!
Download Free
All fields are required.
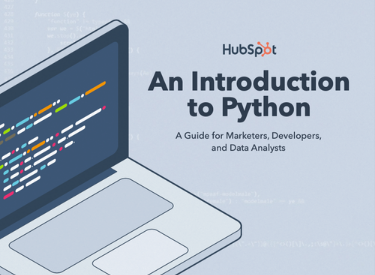