Everyone loves tiny versions of things; that fact holds in programming too.
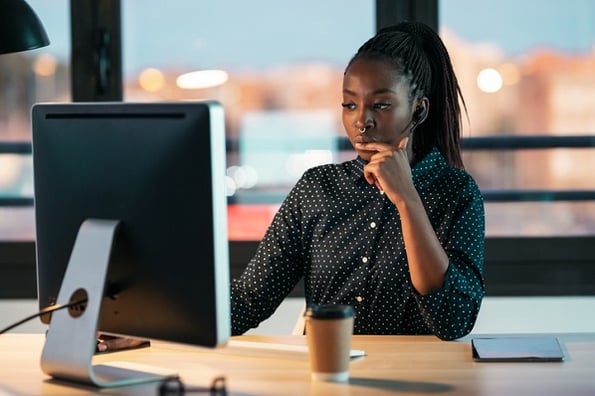
In this post, you will discover the power behind the Python Lambda function and how it works. You'll see some examples and explanations for using the Python Lambda function. Finally, you'll learn how the lambda function can improve your programming.
Let's get started.
What is Lambda in Python
The Python Lambda function is a small anonymous function with only one expression but any number of arguments. When programming, your Python functions may need to be reused throughout the entire software. Moreover, sometimes you will have functions that need access to another one or more times.
Some functions you write will need to be very complex, but that will not always be true. Programs often contain several functions that are more complex than they need to be.
At this point, it may still be a little challenging to understand the improvement the Lambda function truly offers. Check out this awesome video to learn more about how the lambda function works.
Let's take a closer look at some situations that could benefit from a lambda function.
How to Use Lambda in Python
To improve performance, you can split complex functions into smaller reusable pieces that your code can use modularly. One way to do this efficiently is using the Python lambda function, a compact anonymous function.
Breaking your functions into smaller pieces limits the computing load that can come with repeatedly using more complex functions. Moreover, this is also an Object Oriented Programming architecture. In OOP architecture, you create more generalized functions and classes from which other objects can inherit.
A great example of using the Lambda function would be setting up user-level restrictions on features in your software. For example, say you have many user levels — and most software will — you can use a lambda function to perform fast and efficient permission level checks.
Python Lambda Example
There's no better way to solidify coding concepts than to put them in practical use cases. By doing this, you can understand the mechanics of it. Let's look at some examples of how you can use the lambda function to improve your code.
Even if you don't follow the OOP programming architecture, there are still many benefits to the Lambda function. Let's see what using the Python lambda function looks like in some code examples below.
One way to use the lambda function is to pass in two values for any evaluation. Let's look at how you can perform that kind of evaluation modularly.
The code above would result in the number fifty being printed to the console or terminal. This type of function may not seem very useful initially, but you can improve its functionality when you provide more specific information to a lambda function. Let's look at that next.
This lambda function looks misleading as it seems a lot has gone into it, but it's very simple. The code above builds something to interact with so that you can see how the lambda function works. For this example, only two lines need to concern you. Let's dissect this a little further.
This line of code is a simple lambda that accepts an argument to run through a comparison expression.
The line above is a call to the lambda function, passing the required arguments for the function to work correctly. It is important to note that you must pass the values in the correct order. If you do not, the values will be in the wrong places, and the expression will not perform as intended; this is true for any lambda function.
In this code block, the result would be a simple string "Denied" printed to the console or terminal.
You can also use the lambda function within other functions, which can make permission checks easy to make a modular task.
By modularizing this type of task, you can quickly perform it anywhere in your code as often as you need. Lambda functions can simplify your code and reduce repetition for simple functions you will not need to call frequently.
Moving Forward With Lambda Functions
Lambda functions do not seem like much at first glance; however, they provide several benefits, including but not limited to cleaner code. The best way to learn how it can help improve your code is to use them to clean up code.
The best way to identify when you can or should use the Lambda function is to consider how many expressions you will need to run for the task. You may want a standard function if you need more than one expression to run.