When removing elements from a list in Python, the pop() method is your handyman. It’s like a hammer – it may not be perfect for every job, but when you need something removed quickly and efficiently, there's nothing quite like it.
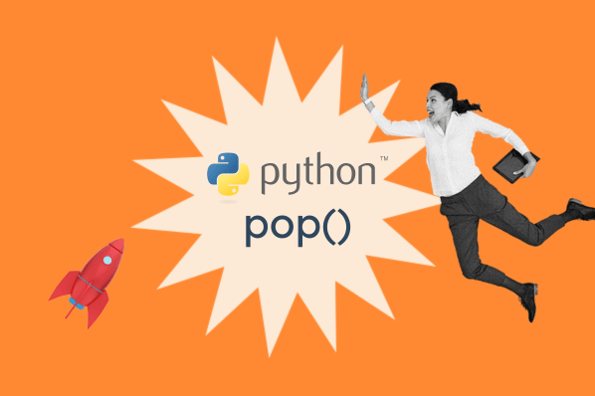
Think of the roles outside of coding - if you had an old refrigerator that needed to go out the door right away, would you call a CPA? Of course not! Instead, you would hire someone with the right tools (and maybe muscles) to take care of it quickly. That’s what pop() does for lists: allow us to remove or "pop" their contents easily. This blog post will explore how the pop() method works its removal magic on any Python list.
What is Python's pop() method?
The pop() method removes and returns the last element from a Python list by default. It can also be used to remove and return an element from a specific position in the list by passing the index of the element to be removed as an argument.
The syntax for the pop() method is as follows:
List is the name of the list you want to manipulate and index is an optional argument indicating the position of an element to be popped. If no index is specified, the last element in the list is removed and returned.
Let's look at an example of a list and how we can use the pop() method to remove and return elements from it:
Removing the Last Element From a List
In this example, the pop() method is used with no argument, which removes and returns the last element in the fruits list ('cherry').
Removing an Element From a Specific Position in a List
In this second example, the pop() method is used with an argument of 2, which removes and returns the third element in the numbers list (3).
In the next section, we will explore how to remove elements from a list using the pop() method, including its behavior when removing elements from a list at specific positions and its return value.
Removing Elements Step-by-step
In Python, a list is a mutable data type, meaning it can be modified after creation. One common operation performed on lists is the removal of elements. The pop() method is used to remove an element from a list, and it does so efficiently by removing the last item from the list by default. However, it can also remove items from other positions in the list if a specific index is specified as an argument.
Let's take a look at how the pop() method is used to remove elements from a list.
Consider the following list:
To remove the last element from the list, we can simply call the pop() method with no arguments, like this:
The pop() method will remove the last element from the list and return its value, which is 5. The updated list will now look like this:
If we want to remove an element from a specific position in the list, we can pass the index of the element to the pop() method. For example, to remove the element at index 1 (i.e., the second element) from the list, we can do the following:
The pop() method will remove the element at index 1 (i.e., the value 2) from the list and return its value. The updated list will now look like this:
Understanding the Behavior of pop() Within a List
It's important to note that when an element is removed from a list using the pop() method, the list is modified in place. This means that the original list is changed, and the element that was removed is no longer part of the list. Furthermore, if the index specified is beyond what is available in the list or it's negative, pop() will throw an IndexError.
It's also worth noting that the pop() method is an efficient way to remove elements from a list because it operates on the end of the list by default. This means it takes constant time to remove an element, regardless of the list size. However, if you need to remove elements from the beginning or middle of a list, the pop() method may not be the best choice, as it would require shifting all the elements in the list after the removed element by one position.
In the next section, we will explore the return value of the pop() method and how it can be used in your code.
Return Value of pop()
The pop() method removes the specified element from the list and returns the removed element. This can be useful if you need to perform further operations on the removed element. If you don't need the removed element, you can simply discard the return value of the pop() method.
This return value can be used in various ways, such as assigning it to a variable or printing it out.
Consider the following example:
In this example, the pop() method is used with an argument of 2, which removes and returns the third element in the numbers list (3). The returned value is then assigned to a variable named third_number and printed out.
If you call the pop() method on an empty list, it will raise an IndexError. Handling this exception is important if you're unsure whether the list contains any elements.
Alternatives to pop()
While the pop() method is useful for removing elements from a list, it's not always the best option depending on the specific use case. There are a few alternatives to the pop() method that can be used in certain situations.
del statement
The del statement can remove an element from a list at a specific index. It's similar to the pop() method with an index argument but doesn't return the removed element.
remove() method
The remove() method can be used to remove the first occurrence of a specified element from a list. It takes the element to be removed as an argument and doesn't return anything.
slicing
Slicing can also be used to remove elements from a list. You can use the slice notation to select a range of elements to keep and create a new list.
In this example, we created a new list by selecting the elements before and after the element to be removed using slicing.
Use Python's pop() Method With Confidence
The Python pop() method is a quick and easy way to remove elements from lists, but understanding its behavior when removing elements from specific positions or their return value can be important for writing efficient code. It's also worth noting that there are alternatives to the pop() method, such as del statements and slicing, which can be used depending on the specific use case. With this knowledge, you can confidently remove elements from Python lists with the pop() method.