Python is a powerful coding language that offers a variety of features, methods, and functions that perform amazing tasks in the software you create. Learning concepts, such as how to replace a character using the Python String Replace method, is a cinch.
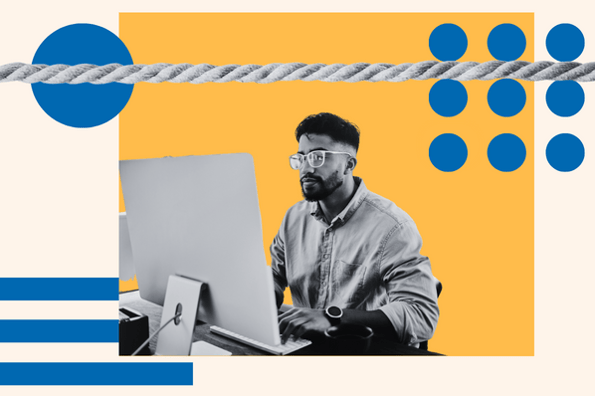
This post will look at Python strings, particularly a method that’s used to make adjustments to them. The method we will cover is called the replace method — we’ll review what it is, what it does, and how it can be useful.
Table of Contents
- Python String Replace Method
- How to Replace a String in Python
- How to Replace Characters in a String Python
- How to Setup Multiple Replacement Rules
- Using re.sub() to Make Complex Rules
Python String Replace Method
Let’s start by looking at the Python string replace method syntax and dissect it into easy-to-manage pieces. The replace method is a method that takes a string and replaces characters within it with new ones. The replace method takes three arguments. Two are required. The third is optional.
string.replace(target, newValue, count)
Breaking this method apart, we can see how it works a little better. The string is a variable that holds a string value. This variable is what we call the method. We chain the method to the variable using a period and pass in the values for the arguments.
Passing a target string will inform the replace method what string to look for while passing in a string for the newValue will replace the target string. Finally, the count argument will determine how many times the replace method will check for and replace characters.
How to Replace a String in Python
Now that we have gone over the components of the string replace method, let’s look at some examples of how it works and discuss its practical uses. We’ll start by looking at a simple example: replacing a word within a string with a new word.
txt = “I love snakes”
newTxt = txt.replace(“snakes”, “my snek”)
print(newTxt)
In the above code example, we have the string “I love snakes” stored in a variable called txt. Then, we call the replace method on that variable and pass in the string we are targeting, as well as its replacement. In this example, we are targeting the string “snakes” from within the txt variable.
As the replace method looks through the string, it will compare all characters with the target we provided; if a match is found, it will replace it with the new value we provided. The result of this process is then saved to the new variable newTxt. Printing this variable will show us the change that we made.
How to Replace Characters in a String in Python
The great thing about this method is that it can also be used to replace individual characters or parts of a word. Let’s look at a few examples of other ways you can use the replace method on strings.
newTxt = txt.replace(“akes”, “eks”)
print(newTxt)
This code does essentially the same thing as our previous example, except in this case, we only gave it part of a word and indicated what we want it replaced with. This is helpful and there are many uses for it, such as fixing spelling errors.
When you incorporate the use of variables and conditions you can create a spell-check system very easily that will check and replace any misspelled words.
newTxt = txt.replace(“s”, “5”)
print(newTxt)
This is still very similar in appearance to the previous two examples. However, this will behave a little differently. Because it is looking for a single character, the likelihood of finding that pattern is higher.
As such, it will check every individual character for any instance of an “s” and replace it with a “5.” When you do not provide a count parameter it will perform this function until the string ends.
Using the Counter Parameter Python Replace String
The third parameter can be thought of as a limiter, as it limits the number of times it will complete this task. If you provide the number one as a limiter/counter, it will only perform the replacement on the first instance of a match.
newTxt = txt.replace(“s”, “5”, 1)
The result of the above code is that the string would be changed to “I love 5nakes,” changing the number to two would result in both “s”s changing to “5”s.
Important caveat: The replace method is letter case sensitive. If the case does not match, it will not be replaced. A lowercase “s” as target means that all uppercase “S”s will be ignored. Only lowercase “s”s will be replaced.
How to Set Up Multiple Replacement Rules
There are multiple approaches to creating multiple replacement rules. The idea of simply using if statements and various conditionals comes to mind. However, an easier approach is to chain together multiple replace methods. Check out the following example.
txt = “Hello”
newTxt = txt.replace(“H”, “J”).replace(“o”, “ow)
print(newTxt)
What do you think the above code will produce? To put what’s happening in plain English, “Hello” is the initial text, but then “H” is replaced with “J,” and then “o” is replaced with “ow.” The final result is “Jellow.” It’s not a real word, but it gets the point across nonetheless.
This process can be repeated as many times as you desire by tacking on additional replace methods, which will then be read from left to right. In other words, the output of each replace method will be fed into the next replace method after it.
If you want to create many replacement rules, it might be better to create a list filled with tuples. Then, iterate over the list, running each rule as you go. Here’s an example of that in action as well.
replacements = [
(“Hello”, “General”),
(“there”, “Kenobi”),
(“!”), “”),
]
txt = “Hello there!”
for old, new in replacements:
txt = txt.replace(old, new)
print(txt)
If you go through the above code, you will get “General Kenobi” as a response (Star Wars, anyone?).
Really, this is no different than chaining together multiple replace methods, but this will certainly look a lot better if you’re doing many replacements at once.
Using re.sub() to Make Complex Rules
If you’re familiar with regular expressions (regex), then you can use this to your advantage in order to make even more complex rules.
To do so, you need to import the “re” module, which is built into Python and contains all things relating to regex in the context of Python. If it’s been a while since you used regex, this cheat sheet might help.
With that said, it’s time to start looking at code.
import re
txt= “Hello, I am actually a cow. Moo!”
print (re.sub(“a”, “y”, txt))
Looking at the above code, what do you think will happen? It should replace “a” with “y”, but there are multiple instances of ‘a’ in the string. Here’s the output:
Hello, I ym yctuylly y cow. Moo!
If you guessed that all instances of ‘a’ would be replaced, good job! However, that might not be the desired result. In order to specify the number of instances you want to replace, the count parameter can be given an argument.
import re
txt= “Hello, I am actually a cow. Moo!”
print (re.sub(“a”, “y”, txt, count=1))
By providing the count parameter with an argument of one, you are telling the re.sub() method to replace only the first instance of “a” with a “y”.
Hello, I ym actually a cow. Moo!
That’s great! Now, you can specify how many instances of the string you want to replace. If you want to get even more specific, regex can be used. For example, you could specify that only ‘a’ characters with white space in front of them should be replaced by using the following code.
import re
txt= “Hello, I am actually a cow. Moo!”
print (re.sub(“\sa”, “y”, txt))
The “\s” is treated as one symbol that represents white space. Therefore, the pattern we are looking for is an ‘a’ character with exactly one unit of white space in front of it.
Hello, I ym yctually y cow. Moo!
Most of the ‘a’ characters in the string have white space in front of them. However, notice that one in the word “actually” has been untouched. With the power of re.sub() and regex, you can create endlessly complex patterns using all sorts of symbols similar to ‘\s’ for anything ranging from alphanumeric characters to unicode to the typically hidden newline characters.
Using the Python String Replace Method in Your Work
Using the Python string replace method is very easy. Let’s review the points we discussed in this post one last time for clarity.
- oldvalue: Required. The string to search for.
- newvalue: Required. The string to replace the old value with.
- count: Optional. A number specifying how many occurrences of the old value you want to replace. Default is all occurrences.
Building on this knowledge will be easy. The more you practice, the better off you’ll be.