Python's remarkable rise as a programming language has been nothing short of spectacular, and it is now the go-to choice for application development and web design.
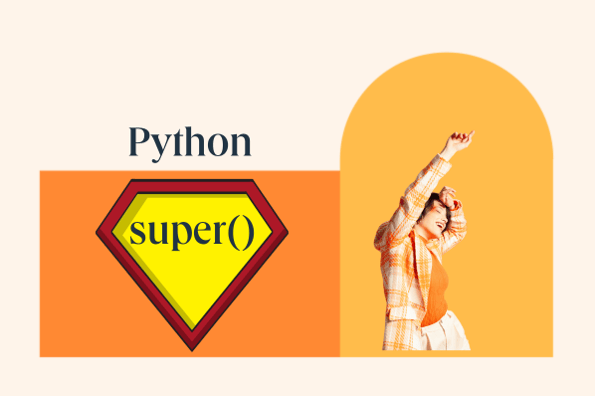
But with all that can be accomplished with Python comes powerful functionality — notably with its super() function — which we'll explore in great detail throughout this guide.
In this blog post, we will explore the Python super() function in-depth, its syntax, and the advantages it offers in inheritance. We will also provide examples to help you better understand its functionality.
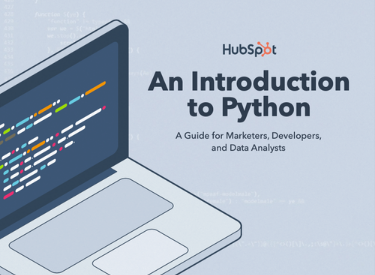
An Introduction to Python
A guide for marketers, developers, and data analysts. You'll learn about...
- What Python is.
- Programming Best Practices.
- Coding Standards.
- And More!
Download Free
All fields are required.
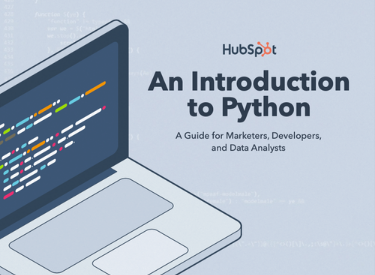
Understanding the Python super() Function
The super() function is a built-in function in Python that provides a convenient way to access and delegate methods and attributes of parent classes. When used, it allows one class to access the methods and properties of another class in the same hierarchy. It is commonly used to avoid redundant code and make it more organized and easier to maintain.
In order to understand how the Python super() function works, we must first understand the concept of inheritance. Inheritance is a powerful feature of object-oriented programming (OOP) that allows a child class to inherit its parent class's methods and attributes without manually coding the same methods or properties in the child class. This makes it easier for us to change our code and makes it more efficient by not having to repeat the same code across multiple classes.
Syntax of super() function
When using the Python super() function, you must include the self-argument in your method. This tells Python that you are referring to the current class, not another class in the hierarchy. See the example below:
In the example, we create two classes — ParentClass and ChildClass — and set them up in an inheritance relationship. Then, inside the ChildClass's init method, we use the super() function to call the init method of its parent class, thus allowing us to access its properties and methods.
The Python super() function can accept two parameters:
- The first parameter is the type of class you are referring to — ParentClass in our example above — and
- The second parameter is the self-argument of the class you are referring to.
Implementing super() Function in Python
When working with the super() function, you must always remember to include the self-argument when calling it — otherwise, the code will not work correctly. With that in mind, let's take a look at some examples of how to use the super() function in Python:
Using the super() Function for Single Inheritance
The super() function is commonly used in single inheritance to call the parent class's methods and attributes from the child class. Here's an example:
In this example, we have a Parent class and a Child class that inherits from the Parent class. The Parent class has an __init__() method that initializes the name attribute, and a greet() method that prints a greeting message.
The Child class has its own __init__() method that initializes the age attribute and calls the Parent class's __init__() method using the super() function. This way, the name attribute is initialized in the Parent class, and the age attribute is initialized in the Child class.
Finally, we create an instance of the Child class and call its greet() method, which uses the name attribute initialized by the Parent class's __init__() method.
As you can see, the super() function makes it easy to call the parent class's methods and attributes without explicitly naming the parent class. In this case, it helped us initialize the name attribute in the Parent class and the age attribute in the Child class.
Using super() Function for Multiple Inheritances
The super() function is also useful in multiple inheritance scenarios where a class inherits from more than one parent class. In this case, the super() function helps ensure that each parent class's methods are called only once and in the correct order.
Here's an example:
In this example, we have two parent classes, Parent1 and Parent2, and a Child class that inherits from both. Both parent classes have a greet() method that prints a greeting message.
The Child class has its own __init__() method that initializes both the name and age attributes by calling the __init__() method of each parent class using the super() function. Note that we explicitly specify Parent2 in the second call to super() to ensure that we call the __init__() method of Parent2 first.
Finally, we create an instance of the Child class and call its greet() method, which calls the greet() method of both parent classes in the correct order.
As you can see, the super() function helped us call the __init__() method of each parent class only once, in the correct order. It also helped us avoid writing redundant code, making it more maintainable.
Accessing parent class attributes using super() function
In addition to calling parent class methods, the super() function can also be used to access parent class attributes. Here's an example:
In addition to calling parent class methods, the super() function can also be used to access parent class attributes. Here's an example:
In this example, we have a Parent class and a Child class that inherits from the Parent class. The Parent class has an __init__() method that initializes the name and age attributes.
The Child class also has an __init__() method that initializes the name attribute using the super() function and initializes the age attribute with a different value. The Child class also has a display_parent_age() method that uses the super() function to access the age attribute of the parent class and prints it.
Finally, we create an instance of the Child class and call its display_parent_age() method, which uses the super() function to access the age attribute of the parent class and prints it.
As you can see, the super() function allowed us to access the age attribute of the parent class from the child class. This is useful when we want to reuse the parent class attributes in the child class but with a different value.
Using super() function with init() method
The __init__() method is a special method in Python classes that are called when an object is created. The super() function is often used with the __init__() method to initialize the attributes of the parent class.
Here's an example:
In this example, we have a Parent class and a Child class that inherits from the Parent class. The Parent class has an __init__() method that initializes the name and age attributes.
The Child class also has an __init__() method that initializes the name attribute using the super() function and initializes the age attribute with a different value.
Finally, we create an instance of the Child class and print its name and age attributes.
As you can see, the super() function allowed us to initialize the name attribute of the parent class in the __init__() method of the child class. This is useful when we want to reuse the initialization code of the parent class in the child class but with an additional initialization code specific to the child class.
Advantages of Using super() Function
The super() function is a useful tool for accessing and reusing the attributes and methods of a parent class in the child class. Here are some of the advantages of using the super() function:
- Method Overriding - The super() function can be used to override the methods of a parent class in the child class.
- Multiple Inheritance - The super() function can be used to access the attributes and methods of multiple parent classes in a child class when multiple inheritances is used.
- Accessing Parent Class Methods - The super() function can be used to access the methods of a parent class in the child class.
- Dynamic delegation of methods - The super() function allows us to delegate methods to a parent class dynamically.
- Solving Diamond Problem - The super() function can be used to solve the diamond problem that occurs in multiple inheritances. The diamond inheritance problem is a common issue in multiple inheritances when a subclass inherits from two parent classes with a common base class.
Final Thoughts on Using the super() Function in Python
The super() function is a useful tool for accessing and reusing the attributes and methods of a parent class in the child class, allowing us to reduce code duplication and improve maintainability. It can be used to access the parent class methods, override them, and solve problems such as the diamond problem that occurs in multiple inheritances.
In this blog post, we've explored how to use the super() function in different scenarios, including with the __init__() method, for single and multiple inheritances, and for accessing parent class attributes. We've also provided code examples to help illustrate each of these scenarios.
By understanding how to use the super() function, you can write cleaner and more maintainable code in your Python projects. It's important to note that the super() function can be complex and requires careful usage to avoid bugs and unexpected behavior.
Overall, the super() function is an important concept to master for anyone working with Python classes and inheritance. It provides a powerful way to reuse and extend code and helps to create well-designed and maintainable code.