File handling in Python is essential. That’s why the programming language has several functions and built-in modules for handling files.
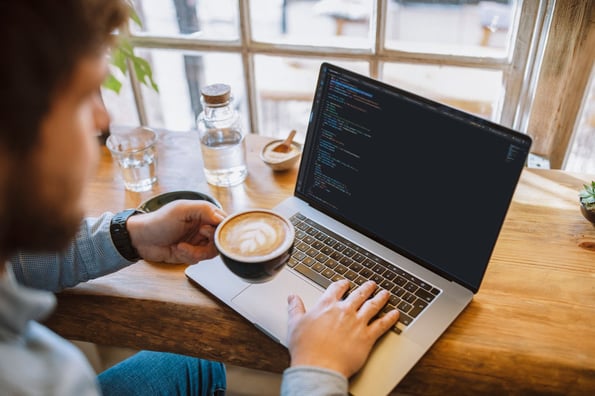
Python offers programmers six access modes to files, and in this article, we’ll show you the code needed to create, read, close, and write files in Python. You’ll also learn about the various file methods every Python programmer needs to be aware of.
In this post, we'll cover:
- What are Python files?
- What is file handling in Python?
- How to Use File Handling in Python
- Python File Handling in Action
Let's get started.
What are Python files?
Files are data or information that exist in the computer’s storage devices. These units of information are used to permanently keep data on a hard disk. By using files, we can keep data for future use.
As a computer user, you certainly know about music files, video files, and text files. Well, Python allows you to manipulate these files.
What is file handling in Python?
Having files is not enough — we need to be able to manipulate them either by opening, closing, writing, or deleting them.
File handling in Python is the process of using Python to carry out one of six operations on files:
- Read Only
- Read and Write
- Write Only
- Write and Read
- Append Only
- Append and Read
In this article, we’ll show you how to manipulate files using Python file-handling methods.
How to Use File Handling in Python
In this section, we’ll show you how to use Python to carry out several operations on a file.
How to Open a File in Python
Python has made opening files quite straightforward because the language has a built-in open function. Reading and writing files is also quite easy in Python. However, before starting any of that, you must first open the files in the right mode. There are three modes available, namely, read, write, or append.
- Read, which is written as “r” in Python, allows you to read the file but doesn’t let you edit or delete any content.
- Write, denoted by “w”, means that if the file exists, all content is deleted before it’s opened to write.
- Append, “a”, opens the file in append mode.
When used, open returns a handle and is instrumental in reading and modifying files. Aside from the three modes mentioned earlier, we can also use the open function to open the file in text or binary modes.
Text mode is the default way files are read and shows strings. Binary, on the other hand, is in bytes and, as such, can only be read by the computer. It’s used for opening non-text files like pictures or program files.
The code snippet shows the different modes of opening files in Python.
It’s a Python best practice to specify the encoding type of your file. This is so because the code might behave differently in different platforms unless the encoding type is specified.
How to Close a File in Python
You should close a file after you’ve completed whatever actions you want. Closing a file in Python is as easy as closing it because Python unsurprisingly offers the close function.
Here’s a code module of how it’s done.
Although opening and closing a file in Python is simple, there are some things you should keep in mind.
- Python could crash if you exceed the limit of the number of files opened at a time.
- Don’t open files that are not useful or usable because this will just make you waste computer memory.
- You should close a file after use to reduce the chances of corruption or data loss.
Furthermore, use the try finally block of code to prevent losing your work. If you don’t use try finally, an exception occurring while performing an operation with the file can cause the code to exit without closing the file.
This code snippet shows how to use the try finally block.
By using this block, the file will close properly even if some exception causes an interruption to the program flow.
Another way of closing a file in Python is by using the with statement. By using this method, we don’t even need to use the close statement.
We recommend this method because it ensures the file closes when the block within the with statement is done.
Python File Handling in Action
In this section, we’ll show you file handling in Python in action. We’ll create a file, open the file, write some random text into it, and pass the file object to the print function.
First, we create the file. To create the file, we’ll use the open() method with an appropriate parameter.
The x parameter will create a file and return an error if that file already exists. The a parameter will create a file if the specified file is non-existent, and the w parameter will create a file as well, as long as it doesn’t already exist.
As an example, let’s use w to create a file called “demo.txt”.
Let’s now write into this new file and close it.
The code above has created a file and written into it. Let’s now read the new file. If correct, it should give us the output “This is a Learning Sample.”
The output is shown below.
Let’s consider another use case of file handling in Python.
We’ll write to an existing file in this example.
The result of this code module is shown below.
In our last example, we’ll delete a file.
To delete a file, though, we have to first import the OS module and run the os.remove() function here’s the code module for that.
Getting Started with File Handling in Python
In this post, you’ve learned how to create, write, read, delete, and close a file using basic Python commands. Now, it’s time to start practicing. Soon, you’ll be an expert at handling files in Python.