HTML is the backbone of the internet. Without it, you wouldn’t have any content. Of course, it takes a lot more than just HTML to create your typical web page, but it definitely builds the foundation for all of the other technologies that go into building a functional website.
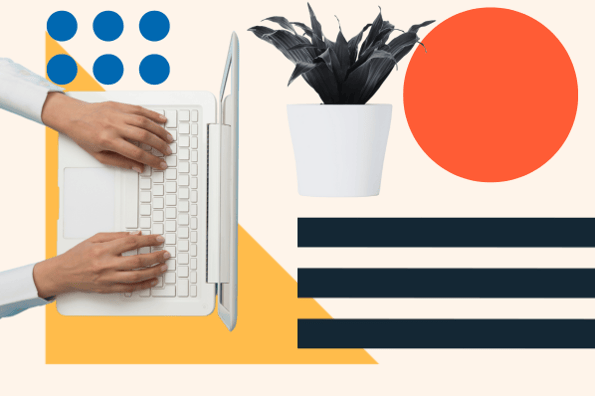
One cool type of functional website uses the concept of tabs. Have you ever visited a website that seemingly loaded pages instantly upon clicking navigation buttons? It’s quite possible that the site used tabs to make that near-instant response happen.
Learn what HTML tabs are and how to add them to your website.
Table of Contents
What are website tabs?
Website tabs are a clever way to show chunks of content on demand at the click of a button. What makes them clever, though, is how they can condense what would be multiple different web pages of content into just one page — without the “one page” part even being apparent to the user. Even more clever, however, is exactly how that is accomplished.
Although there are multiple approaches to this, the site often loads all the content from all the pages at once, then simply hides or displays certain information depending on which tab the user selects. It’s like a magic trick that allows for many single-page web applications to be possible.
In this guide, we’ll focus on the HTML part of the equation, though we’ll briefly touch on CSS and JavaScript as necessary. Some approaches exclude JavaScript, but this guide will use it for simplicity.
HTML Tabs in Action
Let’s take a look at an example of this concept working in action. Here's one from a website ranking football teams:
Each individual box is its own tab that you can click into and expand for more information.
Can you imagine if you had to wait for a new web page to load every single time you clicked one of those boxes? The result would likely be something that feels clunky. Instead, this layout allows you to pack a lot of information onto a single web page without overloading the reader with text and visuals.
Though largely beneficial, there’s one notable trade-off to using this technique. For example, though the tabs “Primary,” “Promotions,” and “Social” are being shown instantly upon getting clicked, they were all loaded simultaneously upon visiting the web page.
This can result in a longer load time upon visiting the web page. However, once past that initial load, everything else is ready to go at a moment’s notice.
How to Create Tabs with HTML
Now, onto the actual task of replicating this effect. As previously discussed, this approach contains HTML, CSS, and JS, though there are different ways to accomplish the same behavior.
1. Create a folder.
To start, create a folder that will contain the files for your site. Then, create empty .html, .css, and .js files inside.
The file names can be anything you want them to be, but keep the file extensions (.html, .css, .js) the same as shown above; otherwise, this will not work.
2. Set up the HTML.
Setting up the HTML itself is actually quite straightforward. We are going to set up a div element to contain our clickable button elements. Then, add a div element for each “page” that we are loading. Each of those respective div elements will then contain content.
These can contain whatever you want, but text elements will be used here for simplicity. See below for an example of how the HTML file might look:
If you not working in an environment like Code Pen, you'll need to include a <link> tag in the <head> of the post to link the stylesheet to your HTML file. You'll also need to add a <script> tag at the end of your post to link your JavaScript to the HTML. These are necessary for the web page to have the basic content without any formatting or functionality.
The CSS link might look like this:
<link rel="stylesheet" href="style.css">
While script element might look like this:
<script src="index.js."></script>
The script element linking the .js file should be at the end of your code. Otherwise, you risk having the script load before your HTML elements, which can break the functionality of your site. In our example, we're going to include the JavaScript directly on the HTML file.
Lines 9-13 contain the buttons you will click on to display content on the page. Ignore the “tab” and “onclick” attributes for now. Just know that’s how we will refer back to these buttons when we get to the CSS and JavaScript portion of the guide.
Lines 15-18, 20-23, and 25-28 are all of the tabs, or pages of content, respectively. Each page of content is contained within its own div element as well as an H2 and a paragraph tag to be displayed on the page.
Again, some simple text was used, but you could put images or videos here. The “id” and “class” properties will again be how we reference these elements later on, specifically in the CSS portion.
Without the CSS portion, all of this HTML would look pretty plain at best and confusing to navigate at worst. So without further delay, let’s move on to the CSS, which is important for formatting and styling our above HTML.
3. Set up the CSS.
The CSS is important for styling and formatting the tabs and their content. CSS property is also used to display or hide the content itself. In the Code Pen below, you can access the CSS file via the CSS tab in the top corner:
Lines 1-6 style the body of the page. In this case, we're using it to style the page's background and to designate the font used throughout the page.
Lines 8-12 style the tab container which is the area of the page that the HTML tab buttons are positioned in. In this case, we're using justify-content and margin-top to center the tabs on the page.
Lines 14-18 apply to all elements that have the “tab” class attribute back in the HTML file, which specifically refers to the div element on lines 10, 11, and 12.
Lines 20-22 affect the actual content on each tab. You'll notice we've set the display property to none. This prevents the tab content from displaying when the page loads, requiring users to click on the buttons in order to view the content. Without this property, you would see the content from all three tabs loaded automatically on the page.
Lines 24-26 style the tab button after it's been clicked on. In this case, I've chosen a dark green to show that the button was clicked, but you can edit the background-color property to be any color you'd like.
Lastly, lines 28-30 will style the actual content of the HTML tab. I've set the display property to block so the content will display itself after the button has been clicked on. You can add additional properties here to further style your tab content.
Now, even with all of that HTML and CSS working together, your web page is still incapable of functionality. If you were to load this up right now, everything would be on the page all at once. To finish the process, you need the power of JavaScript.
4. Set up the JavaScript.
JavaScript is where all of the magic happens. It allows you to change things about your HTML and CSS upon events. In this case, your event will consist of clicking a button with your mouse.
As we mentioned earlier, you can link to your JavaScript file or include it directly within the HTML. This JS code may look something like this:
Line 31 defines a JavaScript function called "openTab" which takes the parameter "tabId." This function will power the tab-switching logic.
Line 33 pulls in all of the elements that have the class "tab-content" and stores them in the variable named "tabContents." This is the content you'll display on your tab and the next line of code hides them from view by setting the display property to none.
The next block of code indicates your active tab. Line 39 stores all elements with the "tab" class in the "tabs" variable. Line 40removes the class "active-tab," applying the proper styling to the correct tag.
Finally, lines 44-47 show the selected tab's content and marks it as active. Line 46 adds the class "active-tab" to the tab that was clicked, marking it as the active tab. It also uses document.querySelector to select the tab based on its onclick attribute.
When all is said and done, open up the index.html file in any web browser, and you should have something like this:
Need a few more tips? Check out this video below to see this process in action.
Building Your First HTML Tab
Now that you’ve made it through to the end of this guide, you can keep building on top of everything that’s been covered. Not only did you learn about the HTML portion of tabs, but also some CSS and JavaScript magic along the way. These are invaluable skills that can be applied in many web projects going forward.