Python has a vast and extensible library that contains a lot of features and packages for different uses. One of those packages is called the requests library, which is used for making API calls. But what is an API and how do developers use them?
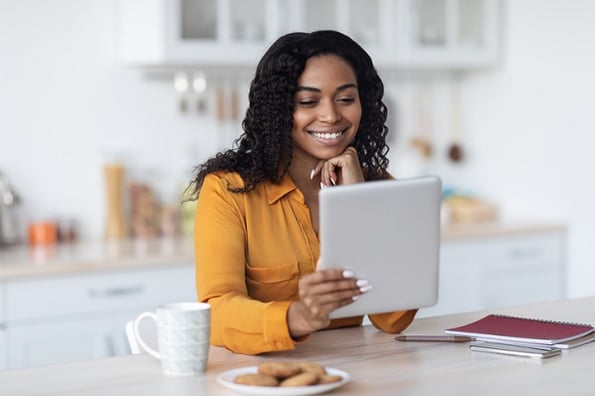
This post will cover how to set up Python to communicate with APIs and how to perform API calls. You will also learn how to use API data and display that data to the users of your software. You will see some code examples showing the syntax and code used to perform these actions to benefit your software.
Without further delay, let’s get started.
What is an API?
The acronym API stands for Application Programming Interface and it is a device such as a server that is used to send and retrieve data using programming code. Most commonly this technology is used to retrieve data from a source and display it to a software application and it’s users.
APIs work in the same way as a browser when you visit a webpage, a request for information is sent to a server and the server responds. The only difference is the type of data that the server responds with, for APIs the data is of the type JSON.
JSON stands for JavaScript Object Notation, which is the standard data notation for APIs in most software languages.
Python API Requests And Response Codes
Any request made to a server of any kind always returns a response object, that response consists of multiple parts. There are two parts of that response that are important for using APIs, one is called the status code and the other is the response body.
The status code is used to indicate the status of the request, such as if it’s succeeded or failed. There is a very large number of response codes that are used to indicate different statuses, let’s look at a few examples.
- 200: Error-free success with a response.
- 301: URL redirect
- 400: Bad request
- 401: Access denied
- 403: Forbidden request
- 404: Not found
- 503: Server error
Each of the response codes starts with a number which is used to help categorize the responses. Responses starting with two indicate success, three indicates a URL redirect, and the numbers four and five mean there was an error encountered.
The requests package is not part of the standard library, so you’ll need to install and import the library for use. To install them using either pip or conda you will need to use the following line of code.
pip install requests
conda install requests
Then to import it you will need to use the following line of code, once you’ve done that you are ready to start making API requests.
import requests
How to Make a Get Request
The process for making requests to an API with Python is actually really simple, you just need to know what API you want to communicate with. In Python you only need a single line of code to make a basic API call, this is done with the get() request function.
Looking at the code below you can see what that process looks like.
response = requests.get("https://someapiurl.clom/api")
This line of code uses the request library to access the get function which then calls the URL of your target. If the URL points to a location that doesn’t exist, has moved or you do not have permissions you will receive a status code indicating the issue. Otherwise, the response object gets saved to the variable response.
To access the data in the response body in a manageable way you can use the Python JSON package which comes as part of the standard library. The JSON package comes with two main functions that you will use to help with data formatting, making it easier to work with the API.
These two functions are the json.dumps() and the json.loads(), which each serve a slightly different purpose. The dumps() function is used to convert a Python object into a string, and loads() take a JSON string and convert it into a Python object. Let’s look at an example of the dumps() function in action, assuming you call the random-user API.
import json
def jprint(obj):
# create a formatted string of the Python JSON object
text = json.dumps(obj, sort_keys=True, indent=4)
print(text)
jprint(response.json())
You might get a response that looks like the following JSON code.
{"results": [{"gender": "male", "name": {"title": "Mr", "first": "Norman", "last": "Knight"}}]}
As you can see this presents a very difficult to read format for the data we want to use, this issue can be dealt with using the JSON dumps() function. The result of passing this object into the dumps() function can be seen below.
{
"results": [
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Norman",
"last": "Knight"
}
}
]
}
As you can see this format is much easier to read and simplifies the process of working with API data. This is all very useful, but what if you want to make a request for data that is more specific, for that you will need to pass in query parameters. There are two ways to do this in Python, using the params keyword or by adding the parameters to the URL.
Adding the parameters via the params keyword is the recommended way to handle queries with parameters. Listing the parameters as a Python dictionary object allows the requests library to handle some of the heavy lifting.
Let’s look at that process next.
How to Make a Request With Parameters
Making a request using parameters is not much different than what you’ve seen thus far, we simply add the params keyword to the get request after the URL. You need to separate the URL from the params keyword using a comma to do this.
Let's see what that looks like next.
parameters = {
"inc": {
gender,
name,
},
"results": 5
}
Using this parameters dictionary we can pass this information into the get() request to specify what data we want from the random user API.
response = requests.get("https://randomuser.me/api/", params=parameters)
Getting Started With Python API
So far you learned a lot about Python APIs, from the theory behind them all the way to the practice of using them. You have discovered how to install the Python requirements for communicating with APIs, and how to use them in your code. You have also learned how to modify requests, and format them for ease of use.
Moving forward you can solidify your knowledge by putting these skills into practice and testing out various open-access APIs. Furthermore, you can build on what you’ve learned here by exploring other parts of the Python requests library.