Are you a programmer that places a counter variable inside a for loop when you need to keep track of iterations? Luckily, the Python enumerate function can handle this task for you.
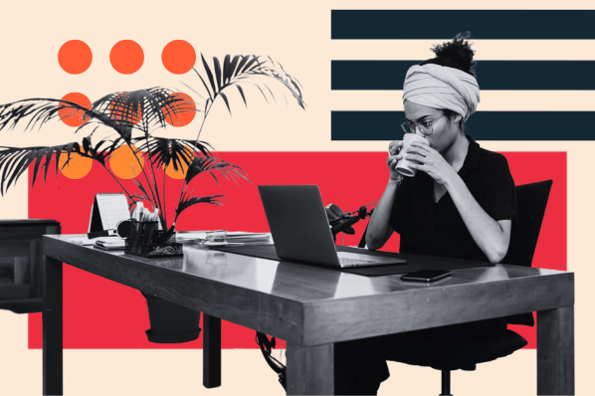
The enumerate() function can make your code easier to read, more efficient, and easier to maintain. In this article, we’ll discuss the Python enumerate function, how to use it, and an example.
Table of Contents
What does enumerate do in Python?
The enumerate function in Python converts a data collection object into an enumerate object. Enumerate returns an object that contains a counter as a key for each value within an object, making items within the collection easier to access. The key is the index of the item.
Looping through objects is useful, but we often need the means to track loops and the items accessed within that iteration of the loop. Enumerate helps with this need by assigning a counter to each item in the object, allowing us to track the accessed items.
This also makes it easier to manipulate our data collections where such a thing is possible. Not all data collection objects in Python are mutable. Sets are a great example of this.
How does enumerate work in Python?
Similar to a for loop, enumerate functions loop over a collection of data once for each item in it. The primary difference is that enumerate also automatically modifies the data it is fed, essentially turning each item into a key-value pair.
As the function iterates over the object, each item is paired with a number corresponding to the number of times the loop has run, including the current loop.
The enumerate function accepts two parameters: the required iterable object and the optional starting number. The starting number parameter accepts an integer and designates what number the function should start counting at. Given that it is optional, the default starting number is always zero, much like the indexing of arrays.
Let’s look at some examples and see how the input is changed by examining the output.
How to Use Enumerate in Python
There are four data types in Python for storing collections of information, but for this example, we will only go over two. The dictionary and list data types are ordered, and therefore, in most situations — barring complex programming like machine learning — would not need to be run through the enumerator function. On the other hand, sets and tuples are unordered and, therefore, more likely to be enumerated.
The Python enumerate function offers a more simplistic way to access and count items in a data collection.
There are four main data collection types in Python. We will look at examples of the enumerate function with the set and tuple. In the next section, we’ll examine these two contrasting but valuable examples using the enumerate function.
Let’s quickly look at the two data types we will use and what sets them apart.
- Tuple: Ordered and unchangeable. Allows duplicate members.
- Set: Unordered, unchangeable (items can be added or removed but not changed), and unindexed. No duplicate members.
Enumerate Python Sets
Python sets are unordered collections of information. The items within them are immutable and, therefore, cannot be changed after they are added. The unordered nature of sets means that the order in which the items within are accessed is inconsistent. They cannot be targeted by an index value, as they do not have one.
The enumerate function associates a counter as a key to each item in the set. This can help create structure in an unordered collection. Enumerating on the set gives us more control over how we can interact with it reliably. This is useful with sets, as items within do not have a designated index location.
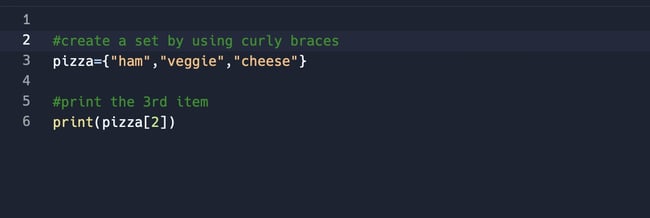
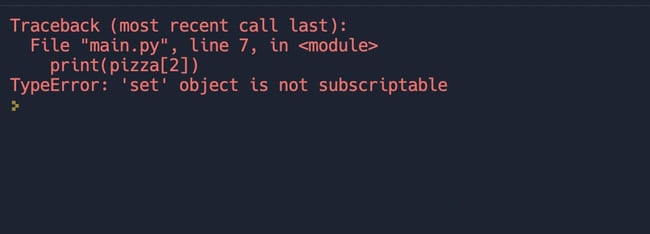
The code above would throw an error — TypeError: ‘set’ object is not subscriptable. This is because targeting an index is incompatible in a set. However, if we enumerate the set, we can use the counter as an index to find our item.
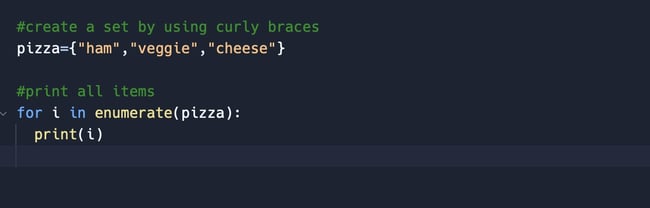
The above code would print to the console the following result. But remember, sets are unordered, so the items returned may vary.
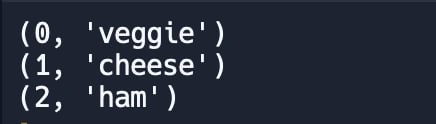
It's important to note that this does not alter the value of pizza. Printing it to the console will give us the same set we declared, likely in a different order.
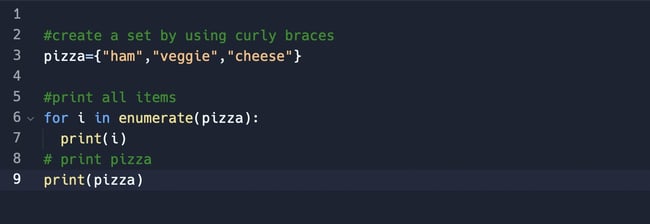
To preserve the effect of the enumerate function on a set, you will need to convert the results to a different data type. Let's look at an example of how we can do that using the list constructor function.
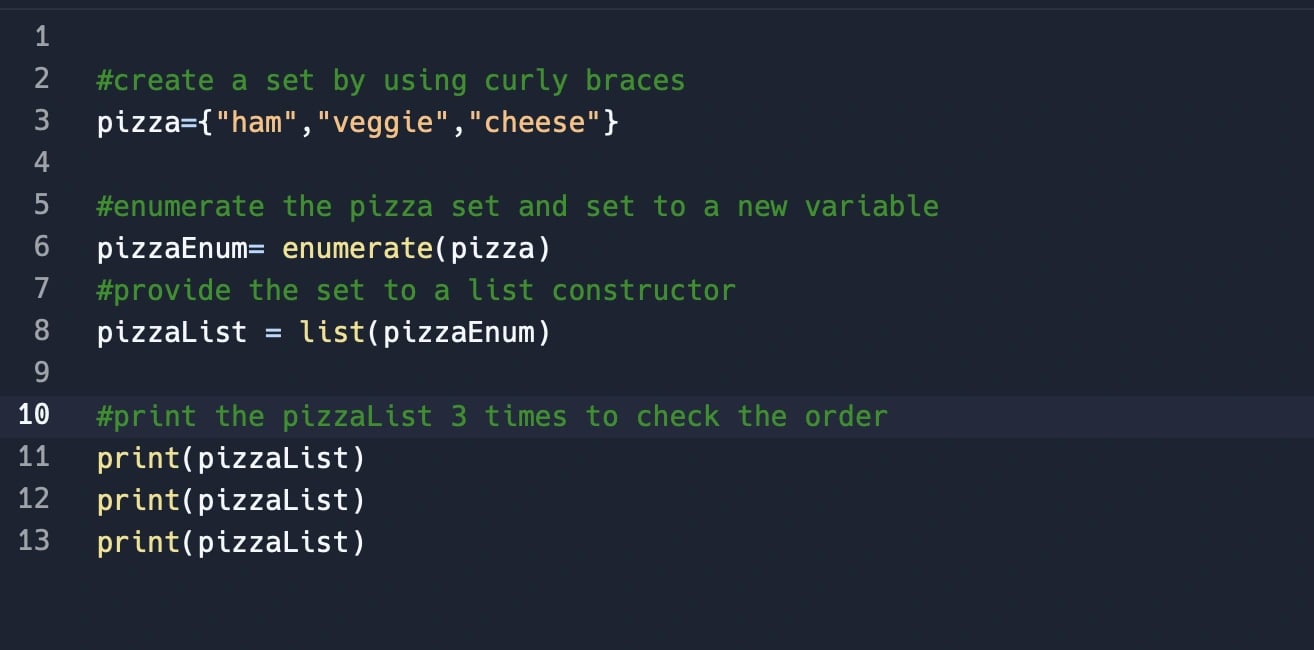
First, we create the set. Then we run the enumerate function providing our set and save that to a new variable. Finally, the result of the enumeration is then provided to the list constructor and saved as a new list object. The data inside of this new setlist object would look like a list of key/value pairs.
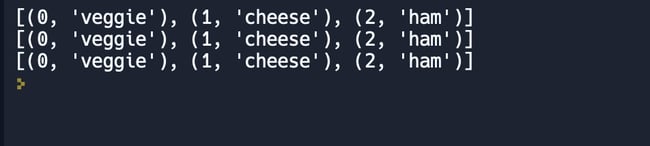
Enumerate Python Tuples
The enumerate function can also be used on tuples to get key/value pairs. This comes with much more reliability regarding the order in which items will be returned.
Tuples are ordered, which means items within are in the position at which they were added. So, the second item in a tuple — unlike a set — can be targeted using the bracket notation with the index of 1.
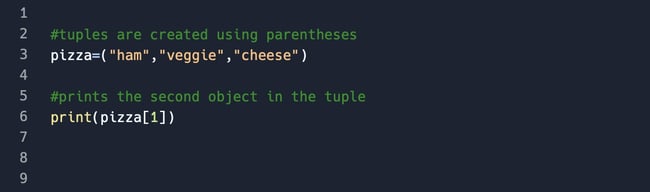
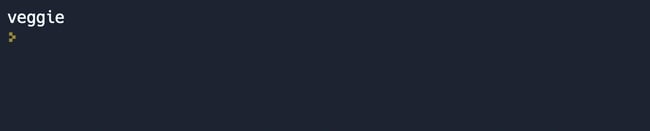
Enumerating Python tuples is more straightforward than enumerating sets. Enumerating over tuples in Python has far more predictable and consistent results. Let's look at an example of how we would do this.
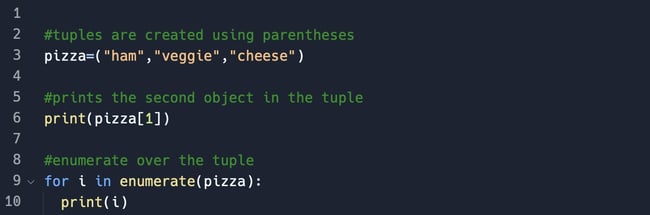
For each iteration of the loop, the result will be printed to the console showing the enumerated keys and values for the snekTuple we created.

It is important to note that this example is a little redundant, as tuples are ordered and can be targeted using indexes. That isn‘t to say that there isn’t a viable reason or use for this.
A great example is enumerating over a dictionary of sets. The sets within the dictionary could be enumerated to better understand its contents.
Getting Started with the Python Enumerate Function
There are a lot of useful tricks that can be applied to your programming with the enumerate function.
The complexity of your programming will impact how often you'll need features like the enumerate function. Enumerate will also affect how complex the use of such features will be, and the enumerate function can be used to perform powerful data restructuring for a variety of needs.
Best of luck, and be sure to utilize resources like Stack Overflow and the Python documentation page as needed.
Editor's note: This post was originally published in March 2022 and has been updated for comprehensiveness.