Experienced programmers agree that reading quality code samples can influence how quickly and effectively you’ll learn Python. At the start of your journey, you’ll likely encounter methods with SELF.
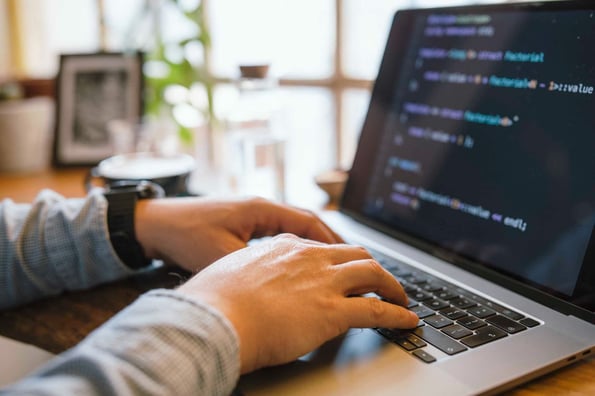
In this post, you’ll learn about the SELF parameter in Python. That includes why it’s used, its definition, and variable initialization. Then, you’ll see how to use SELF.
More specifically, we'll cover:
- What is SELF in Python?
- What are instance and class methods in Python?
- When to Use Self in Python
- Python Self in Action
Let's get started.
What is SELF in Python?
SELF represents the instance of class. This handy keyword allows you to access variables, attributes, and methods of a defined class in Python.
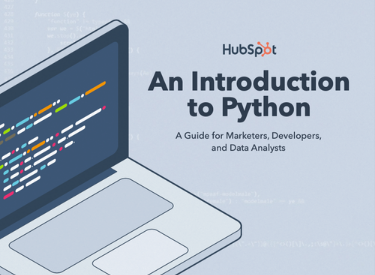
An Introduction to Python
A guide for marketers, developers, and data analysts. You'll learn about...
- What Python is.
- Programming Best Practices.
- Coding Standards.
- And More!
Download Free
All fields are required.
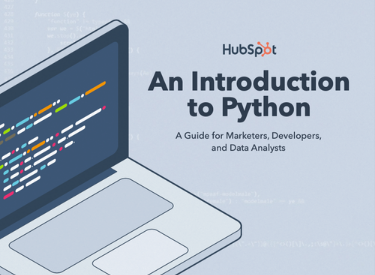
The self parameter doesn’t have to be named “self,” as you can call it by any other name. However, the self parameter must always be the first parameter of any class function, regardless of the name chosen. So instead of self, you could use “Mine” or “Ours” or anything else.
Despite the definition above, we understand that what SELF really means is still hazy. To thoroughly understand what SELF is, you need to understand two basic Python ideas: instance methods and class methods.
What are instance and class methods in Python?
You might have encountered the term “instance method” as you learn Python. They are one of the fundamental building blocks of writing Python code.
These methods can access instances of a class and are used to read or write instance attributes. Understanding them will help you understand what SELF in Python is.
The first step in creating an instance method is to create a Python class. And you do this by using the “Class” keyword.
Once you’ve created the class, you can define an instance method in the class by using a similar syntax as what you’d use to define a function.
Let’s call our class Fruits and create an instance method in it.
Here are the logical steps in the code above.
- First, we define a class called Fruits.
- Next, we added the class constructor (_init_). This class constructor allows us to create instances of Fruits that have two attributes, namely, color and type.
- Finally, we define an instance method (describe).
The instance method must have the first argument as SELF. This argument is the instance of the class that the instance method is called on.
SELF is thus an instance of a class (or object), and we’ve used it in two methods of the Fruits class.
First, we used it in the _init_() method. It’s used here to update the state of the instance of the class.
Secondly, we used it in the describe() method. It’s used here to read the values of the attributes type and color so that we get a print statement. In other words, it reads the state of an object.
When to Use Self in Python
Now that we know what self in python means, when should you use self?
Whenever a class is made in Python, the coder needs to have a way of accessing the attributes and methods of the said class. Other programming languages have a fixed syntax assigned to refer to these attributes and methods. For example, the C++ language uses the “this” syntax.
Python, on the other hand, uses SELF as the first parameter of methods representing the instance of the class. Aside from that, SELF can also represent a variable field within the class.
Let’s consider an example.
Self is the name variable of the MyName class. So if there is a variable within a method, SELF won’t work.
So SELF should be used when you define an instance method, as it’s passed automatically as the first parameter when the method is called.
You should also use self in Python when referencing a class attribute from inside an instance method.
SELF also comes in handy when you refer to instance methods from other instance methods.
Python Self in Action
Consider the code below:
“Def” has an attribute and holds a function. When a function is defined within a class, it’s known as a method.
So, in the example above, “def sample_method” adds the content of “sample_method” into the DemoClass class.
When we run the code, we get an output of all the members of the DemoClass. The method “sample_method” is also included.
Let’s now consider a similar piece of code, albeit with slight differences.
The code has the same class with the same method. However, we now have a constructor program statement denoted by “the_object” in the code.
Running the code gives the same results because “the_object” is still based on the “DemoClass” class. However, the “sample_method” is now the method of the object that the name “the_object” is pointing to.
Are you following so far? Good, because things are about to get funny.
Let’s modify our code even further.
We have the same class, “DemoClass” and “sample_method” in this example. However, the last line now has what is called a dot notation.
The last line of code is the_object.sample_method(). In this line of code, the_object is the name bound to the object. sample_method is the name of the method inside the object. So what this line of code is doing is it asks the “print” line of code to execute. It messages the object, where the object is an instance of DemoClass.
Although the code looks good and we expect to see “This is the demo method” printed, running it actually results in an error message, as seen below.
Python tells us that “sample_method takes 0 positional arguments, but 1 was given”.
Well, we certainly haven’t put any arguments in the “def sample_method()”, as the “()” is empty, so that is correct. But the error says, “1 was given”. A look at the last line of code also shows that the () is also empty! How can you solve this error?
By using SELF!
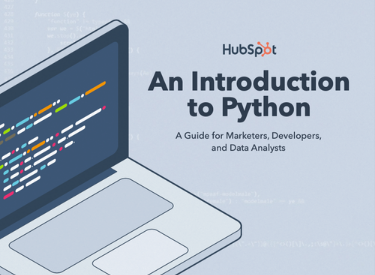
An Introduction to Python
A guide for marketers, developers, and data analysts. You'll learn about...
- What Python is.
- Programming Best Practices.
- Coding Standards.
- And More!
Download Free
All fields are required.
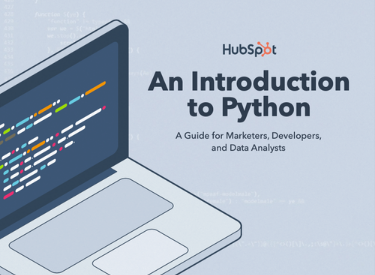
Take a look at the code module below.
Notice any changes? Well, we have passed SELF into “sample_method”. Running the program now doesn’t return any errors, and our code runs perfectly!
Getting Started with Python Self
Self in Python is one of the most used conventions by Python developers. Although you can use literally any other word instead of SELF as your first parameter, using SELF improves the readability of code. We hope this article has improved your understanding of this important aspect of Python.