Python is one of the most sought-after programming languages nowadays because it allows developers to focus on implementation rather than spend time writing complex programs. The ease of access and readability makes Python ideal for beginners who want to learn the basics of coding. To advance as a Python programmer, you need to understand the basics of variables.
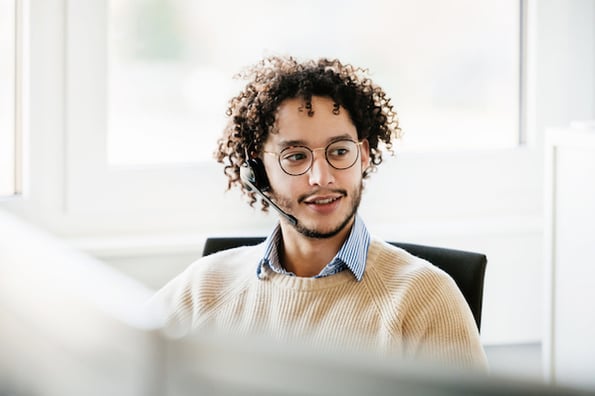
In this article, we will explore what variables are in Python and how to create them. We will also discuss best practices and common mistakes people make when working with variables. By the end of this article, you should have a strong understanding of how variables work in Python. Let's get started.
What is a variable in Python?
Python variables are like containers that hold values. They can be used to store anything from simple data types like integers and strings to more complex data structures like lists and dictionaries. You can think of them as labels you attach to values to keep track of them.
Variables are useful because they can help you keep track of different pieces of information in your program. For example, if you were writing a program to keep track of your expenses, you might want to use variables to store the amount of money you spent on each purchase. This would be much easier than remembering all the different values yourself.
Next, we will look at different ways to form a variable. Let's begin with discussing how to name a Python variable.
Name a Variable in Python
When naming variables in Python, there are a few rules that you need to follow:
- Variable names can only contain letters, numbers, and underscores.
- Variable names cannot start with a number.
- Variable names are case sensitive (my_variable is different from My_Variable).
There are also a few conventions that people follow when naming variables:
- Variable names should be short but descriptive.
- Variable names should be all lowercase.
- If you need to use multiple words in your variable name, you can separate them with underscores. Example: my_very_long_variable_name = 42.
Remember to be as clear and concise as possible when naming variables. This will help make your code easier to read and understand.
Some examples of good variable names:
- my_variable
- var_name
- num_of_items
- user_input.
Some examples of names you should avoid:
- myvariable
- vname
- numitems
- input
Create a Variable in Python
Creating a variable in Python is simple. Just use the assignment operator (=) to assign a value to a variable.
Consider the following example:
In the code example, we will create a variable called my_variable and assign the value 42 to it. You can then use this variable in your code by referencing its name:
The code above prints the value of my_variable to the screen.
Declare a Variable in Python
Unlike other programming languages; Python does not require you to declare variables explicitly before using them. This means you can create a variable and start using it without any declaration. Since Python is a dynamically-typed language, variables are created when you first assign a value to them.
Take a look at the following example:
This code will create the variable my_variable and automatically set its type to int (integer).
Note: String variables can be declared using either single or double quotes.
Example:
Reassign a Variable in Python
You can reassign values of different types to the same variable without issue.
Take a look at the following example:
Now my_variable will be of type str (string). This is one of the benefits of using a dynamically-typed language - you don't need to worry about declaring variables ahead of time; you can just start using them.
Assign Multiple Variables at Once
In Python, you can assign multiple variables at the same time. This is often useful when extracting multiple values from a data structure, like a list or dictionary.
Review the following example:
In this code we assign the value 0 to the variable a, and the value 42 to the variable b. You can also use this syntax to swap the values of two variables:
This will take the value of b and assign it to a, and vice versa.
You can also assign multiple variables from a list:
This will assign the first value in the list ( 0 ) to a, the second value ( 42 ) to b, and the third value ( "hello" ) to c.
Note: This only works if the number of variables being assigned matches the number of values in the list. Otherwise, you will get an error.
Get the Type of Variable in Python
It's often useful to know the variable type in Python. You can use the type() function to find out:
This would return int, str, list, dict, tuple, etc., depending on the type of the variable.
Take a look at the example below:
You can also use the isinstance() function to check if a variable is of a certain type:
This would return True or False depending on whether or not my_variable is a string.
Remove a Variable in Python
If you want to remove a variable in Python, you can use the del keyword.
For example:
This will remove the variable my_variable from your namespace. After running this code, trying to access my_variable will result in an error.
You can also remove multiple variables at once by using the del keyword with a list of variable names:
This will remove both my_variable and my_other_variable from your namespace.
Generally, you won't need to delete variables very often. However, there are a few situations where it can be useful:
- When you are finished with a large data structure and want to free up memory
- When you want to make sure a variable can't be accessed or modified anymore
- When you want to avoid potential name collisions with new variables
Keep in mind that once you delete a variable, it is gone for good. There is no way to recover it, so make sure you only delete variables that you no longer need.
Local and Global Variables in Python
When working with variables in Python, you need to be aware of the scope of the variable. The scope of a variable is the part of your code where the variable is available. When you create a variable in Python, it is either a local or global variable.
A local variable is only available within the scope in which it is created. For example, if you create a variable inside of a function, it will only be available inside of that function.
A global variable is available throughout your entire Python program. For example, if you create a variable at the top level of your program (outside of any functions), it will be globally available throughout your program.
Simply stated, if you wish to use the same variable throughout your program declare it as a global variable; whereas, if you want to utilize that variable in only one function or procedure of your code, declare it as a local variable.
Note: You should generally avoid using global variables in your code, as they can lead to unexpected results and make your code difficult to debug.
Below is an example of a global variable:
In this example, the variable my_var is declared as a global variable. This means that it can be accessed from anywhere in your program. In the function my_func(), we simply print the value of my_var to the screen.
Now, let's say we wanted to create a local variable inside of the function instead:
In this example, the variable my_var is declared as a local variable inside of the function. This means that it can only be accessed from within the function. If we try to access it outside of the function, we will get an error:
This would cause an error because the variable my_var is not available outside of the function.
Using the global keyword, you can also declare variables as global inside of a function.
Take a look at the following example:
In this code, we use the global keyword to declare the variable my_var as a global variable inside of the function. This means that we can access it from anywhere in our program, even outside of the function.
This wraps up the several different ways to form a variable. Next, we will discuss some common mistakes to keep in mind.
Common Pitfalls when Working with Variables in Python
There are a few things to watch out for when working with variables in Python. Let's take a look at some of the most common mistakes people make.
Mistake #1: Not Assigning a Value
One common mistake is trying to use a variable before it has been assigned a value.
Take a look at the following example:
This would cause an error because my_variable has not been assigned a value yet. You would need to do something like this first:
This will print None to the screen, which is the default value for variables that have not been initialized with a specific value.
Mistake #2: Reassigning a Constant Value
Another common mistake is trying to reassign a constant value. For example, you might see code like this:
The example above would cause an error because PI is a constant value (it is set to math.pi by default) and cannot be changed.
A constant value is a value that cannot be changed. In Python, there are a few built-in constants, such as None, True, and False. You can also create your own constants by using the constant module. The constant module is a module that allows you to create your own constants in Python by using the import keyword.
If you want to reassign the value of PI, you would need to do something like this:
This would reassign the value of PI to "Hello, world!" in the math module.
With that said, constants are not really used in Python that often, so you probably won't run into this issue very often.
Mistake #3: Using Undeclared Variables
Another common mistake is using an undeclared variable.
Let's look at an example:
This will cause an error because the variable x has not been declared yet. You would need to do something like this first:
This will declare the variable x and assign the value 42 to it, then print the value of x to the screen.
Hopefully this has cleared up some of the confusion around variables in Python.
Final Thoughts on Variables in Python
Variables are an essential part of programming in any language. Overall, Python variables are simple to create and use. Just remember to choose meaningful names, and don't delete any variables that you might need later. Also, be careful of the variable scope when working with functions.
Now that you know all about variables in Python, you're ready to tackle some other important concepts in Python programming!