Similar to HTML tags and Javascript reserved words, Python has special keywords that you can use to execute tasks. There are several built-in to the programming language and they perform a range of functions from verifying lines of code to importing new modules.
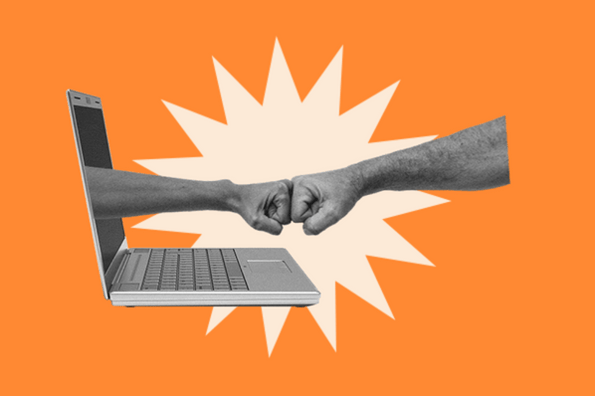
![Download Now: An Introduction to Python [Free Guide]](https://no-cache.hubspot.com/cta/default/53/922df773-4c5c-41f9-aceb-803a06192aa2.png)
In this post, we provide a brief overview of what a Python keyword is and how to form a Python statement. Then dive into the ultimate list of Python keywords including interactive examples you can use to kick-start your programming.
What is a Python Keyword?
A Python keyword is a word that serves a specific function in Python. It is limited to one single function and it can not be set as a variable name, a function name, or the value of any other identifier. The purpose of the keyword is to define the syntax of the code.
All Python keywords -- with the exception of True, False, and None -- are written in lowercase and are case-sensitive.
What are Python identifiers?
Python identifiers provide a name for methods, classes, and variables. Identifiers are also case-sensitive and can not be named after a keyword. If you're worried about that limiting your options, you can use an underscore (_) to combine words and/or numbers into one identifier.
In the example below, the identifier is the word "Dog_Types."
Dog_Types = 'Dog Breeds'
What are Python statements?
When you combine keywords together, you can create statements. Statements are instructions for the program to execute.
Here's a simple example using the "try" and "except" keywords.
try:
print(x)
except:
print("try example")
In this example, the try keyword is testing the code for errors while the except keyword fixes any errors that are found. The output for this code would be: try example.
All Python Keywords & Statements
def | with | return | finally |
if | not | pass | yield |
for | or | and | await |
try | del | as | async |
except | break | is | nonlocal |
class | import | from | global |
in | True | raise | assert |
while | False | continue | lambda |
The links above will help you navigate to specific keywords. Use this list as a handy reference doc to hone your programming skills, learn new syntax, and become a Python expert.
1. def
In Python, a function is defined or declared using the def keyword.
Definition Keyword Example:
def my_function():
print("Hello from a function")
Output:
Hello from a function
2. if
In Python, an if function (or statement) runs an expression only if certain conditions are met.
If Statement Example:
x = 10
y = 10
if x == y: print("x is equal to y")
Output:
X is equal to Y
3. for
The for keyword creates loops that can iterate over an iterable object. This looping method allows us to execute a set of code multiple times with different values each time.
For Keyword Example:
for i in range(5):
print(i)
Output:
0, 1, 2, 3, 4
4. try
The try function or statement tests a code for errors.
Try Statement Example:
try:
print(x)
except:
print("try example")
Output:
try example
5. except
The except keyword fixes errors or "exceptions" in Python. It is typically used with the try block.
Except Statement Example:
try:
print(x)
except:
print("except example")
Output:
except example
6. class
In Python, you can create a custom class using the class keyword.
>> Learn more about Python classes
Class Keyword Example:
class NewClass:
myvalue = 10
print(NewClass)
Output:
<class '__main__.NewClass'>
7. in
The in keyword is used to check if a sequence contains a specified value. It can be used with strings, lists, tuples and dictionaries.
In Keyword Example:
my_list = ["a", "b", "c"] if "a" in my_list:
print("The list contains a")
Output:
“The list contains a”
// This statement will print “The list contains a” because the in keyword checks if the value “a” is in the list.
8. while
The while keyword creates loops that can iterate until a given condition is no longer true. This looping method allows us to execute a set of code multiple times while a condition is met.
While Keyword Example:
i = 0 while i < 5:
print(i) i += 1
Output:
0, 1, 2, 3, 4
9. with
The with keyword is used to create a context manager. This allows us to ensure that resources are properly managed, such as closing files or releasing locks.
With Keyword Example:
with open("file.txt") as f:
print(f.read())
Output:
// This statement will print the contents of file.txt to the console.
10. not
The not keyword is used to reverse the boolean value of a statement. It can be used to check if a condition is false instead of true.
Not Keyword Example:
x = 2 if not x == 3:
print("x is not equal to 3")
Output:
“x is not equal to 3”
11. or
The or keyword is used to check if either of the conditions evaluated is true. If either condition evaluates to True, then the expression returns True.
Or Keyword Example:
x = 5 y = 10 if x > 3 or y < 15:
print("One of the conditions is true")
Output:
“One of the conditions is true”
12. del
The delete or del function is used to delete objects in Python.
Del Keyword Example:
d = {"name": "John", "age": 24}
del d["name"]
print(d)
Output:
{'age': 24}
13. break
The break function or statement in Oython is used to terminate an active loop. It takes a list of numbers as an argument and returns the average of all the values in the list.
Break Statement Example:
for i in range(1, 10):
if i == 5:
break
print(i)
Output:
1,2,3,4
//Thisstatementwillprint1,2,3,4becausetheloopisterminatedwheniisequalto5.
14. import
The import statement is used to import modules or packages in Python. It allows us to use the functionality defined in those modules in our code.
Import Statement Example:
import math
print(math.pi)
Output:
3.14
// This statement will print the value of pi because it imports the math module and then prints the value of pi using the math.pi statement.
15. True
The True keyword is used to evaluate a comparison. It is typically used as an output value in a boolean expression.
True Keyword Example:
print(1 is 1)
Output:
True
16. False
The True keyword is used to evaluate a comparison. It is typically used as an output value for a boolean expression.
True Keyword Example:
print(1 is 3)
Output:
False
17. return
The return keyword is used to define functions that will return a value. This can be useful when you need to perform a specific operation and return the result.
Value Returning Function Example:
def sum(a, b):
return a + b
print(sum(4, 5))
Output:
9
// This statement will evaluate to 9 because the sum of 4 and 5 is 9.
How to Resolve Syntax Error 'Return' Outside Function
The syntax error 'return' outside function occurs when a return statement is used outside of a function definition in python. A python function must be defined before a return statement can be used.
Incorrect Example:
return 1
// This statement will result in a python syntax error 'return' outside function because the return statement is not within a Python function definition.
Correct Example:
def func():
return 1
// This statement will not result in an error because the return statement is within a Python function definition.
18. pass
The pass statement is a placeholder for future code.
Pass Function Example:
def my_func():
pass
print("This will be printed!")
Output:
This will be printed!
How to Pass a Variable to Function
Python functions can take arguments in the form of variables. These variables can be passed to a python function as parameters and used within the function.
Example:
def my_function(arg1, arg2):
print(arg1 + arg2)
my_function(1, 2)
Output:
3
19. and
The and keyword is used to combine two or multiple statements together into one condition.
and Keyword Example:
a = 'apple'
if 3 > 1 and 2 < 4 and 2==2:
print(a)
Output:
apple
20. as
The as keyword gives an alias to a module upon import. You can then refer to the module using a user-defined name.
as Keyword Example:
import math as trig
print (trig.sin(10))
Output:
-0.5440211108893698
21. is
The is keyword determines if too items or variables refer to the same object.
is Keyword Example:
Love = 1
Love is Love
Output:
True
22. from
The from keyword imports a specific section of a module.
from Keyword Example:
from math import sin
print(sin(10))
Output:
-0.5440211108893698
23. raise
The raise keyword is used to raise an exception.
raise Keyword Example:
def my_func(x):
if x < 0:
raise ValueError("x cannot be negative")
Output:
ValueError: x cannot be negative
// This statement will raise a ValueError if the value of x is negative.
24. continue
The continue keyword is used to skip the remaining part of a loop body and return to the top of the loop.
continue Keyword Example:
for i in range(10):
if i == 5:
continue
print(i)
Output:
0,1,2,3,4,6,7,8,9
25. finally
The finally keyword is used to define a block of code that will be executed regardless of whether an exception occurs or not.
finally Keyword Example:
try:
x = 1 / 0
except Exception:
print('Error Occured')
finally:
print('Finally Block Executed')
Output:
Error Occured
Finally Block Executed
// This statement will print 'Error Occured' and then 'Finally Block Executed' because the finally block is executed regardless of whether an exception occurs or not.
26. yield
The yield keyword is used to generate a sequence of values. It allows a function to provide values one at a time.
yield Keyword Example:
def my_func():
yield 1
yield 2
for num in my_func():
print(num)
Output:
1 2
27. await
The await keyword is used to wait for an asynchronous function to complete before continuing with the program.
await Keyword Example:
async def my_async_func():
await some_async_task()
Output:
// This statement will wait for the some_async_task() to complete before continuing with the program using the await keyword.
28. async
The async keyword is used to define an asynchronous function in Python.
async Keyword Example:
async def my_async_func():
await some_async_task()
Output:
// This statement will define an asynchronous function that awaits the completion of some_async_task() using the async keyword.
29. nonlocal
The nonlocal keyword is used to declare a variable as an outer scope of the current function.
nonlocal Keyword Example:
def my_func():
x = 0
def inner_func():
nonlocal x
x += 1
print(x)
inner_func()
Output:
1
30. global
The global keyword is used to declare a variable as a global scope.
global Keyword Example:
def my_func():
x = 0
def inner_func():
global x
x += 1
print(x) inner_func()
Output:
1
31. assert
The assert keyword is used for debugging purposes. It checks if a condition is true, and if it is not, it will raise an error.
Assert Keyword Example:
x = 3
assert x > 2
print(x)
Output:
3
32. lambda: lambda arguments : expression
The lambda keyword is used to create anonymous functions (functions that are not bound to a name). These functions are commonly used to simplify code.
Lambda Keyword vs. Python Function
The lambda keyword is an anonymous Python function that takes any number of arguments and returns the value of a single expression. The lambda syntax is formatted like so: lambda arguments : expression.
On the other hand, Python functions are user-defined functions that take multiple parameters and execute code statements. They are declared with the keyword def followed by their name and a list of parameters.
>> Learn more about the lambda function here.
Lambda Keyword Example:
sum = lambda x, y : x + y
print(sum(4, 5))
Output:
9
// This statement will evaluate to 9 because the lambda function adds 4 and 5 together.