Typecasting is a process many developers don't give much thought to, but at some point in your software development journey, you will typecast one data type into another.
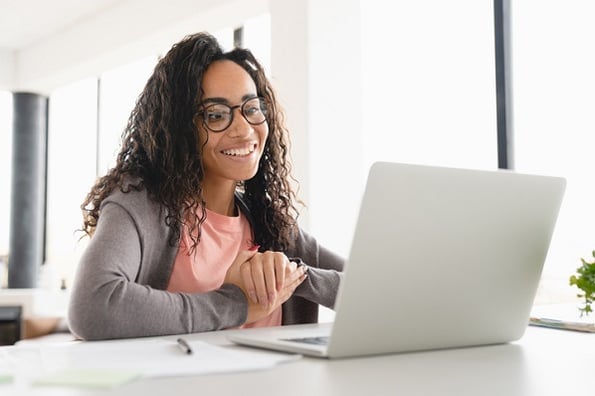
This post will cover Python typecasting and how it is performed. You will also learn how to typecast strings, particularly how to typecast strings into integers. This process can be constructive when you need to ensure specific data types are processed correctly. You will also see examples of why typecasting is essential and why you might end up using it in your code.
Without further ado, let's slither right in.
Why Typecast str to Int
You may find yourself in many different situations as a developer that require converting data types into another type. An example would be casting strings into numbers and casting numbers into a string. Casting an int to a string is more accessible than the alternative, as any number can be a string, but only numbers can be an int.
Python offers tools that can help facilitate this process and simplify your workflow. Typecasting is a helpful skill every developer will use at some point in their development journey. In the section below, let's look at how you can perform this task.
How to Convert str to Int in Python
As mentioned above, you can easily cast any int value into a string. However, this does not go both ways. Casting strings to ints is a little trickier and must be done carefully. Trying to cast a non-number string into an integer will likely result in undesirable behavior. Check out this next video to learn more about how the typecasting process works.
To help facilitate this process, Python offers the int() function, which can take a string number and cast it as an int for you. This function helps alleviate several potential problems, including accidentally performing arithmetic operations on strings which will undoubtedly cause errors in your code.
With the int() function, you can pass in a string number, which will do the heavy lifting. Let's take a look at that next.
That's a straightforward function that takes a string number and converts it into a string. Printing the variable's value in the code above would result in the int seven printed to the terminal or console.
One way to check if your string or int is of the desired type is to use the type() function, which takes a value and determines its data type. For example, passing in the variable above would cause the type() function to return the data type, which, when printed to the console, would show the text below.
Confirming that the string passed into the int() function was successfully typecasted to an int. Now that you have learned how the typecasting process works from str to int, let's look at a couple of examples of how this can be a helpful task.
Convert str to int Examples
When developing software, you will often require information from the users of your application, information that, when collected from the user, will always be in the form of a string. But if you want to work with numbers — determining the amount of time that has passed — you will need a way to change that string to an int.
Let's look at how you can perform that task in the code below.
The code above prompts the user to provide a response which is then collected as a string, which you can set up in the required format. Then you can convert that string into an int or collection of ints you can use to perform calculations. Let's look at how you can do that with the year a car was made.
The code above successfully prompts the user for information regarding the vehicle year and then converts that input from a string to an int. It's that simple and can help you control the flow and type of data that moves around in your application. Controlling the type of data passed around is as important as managing how it gets passed around.
Moving Forward With Python String to Int Typecasting
You've learned everything you need to know to start your journey with string to int typecasting in Python. Armed with the information provided in this post, you can start moving forward with typecasting and putting this knowledge into practical use.
As always, the best way to solidify new information is with practice, create strings, and practice casting strings with numbers into ints. Practice is the best way to learn the performance boundaries of typecasting and discover the nuances of this task. Another way to move forward is to find ways to slice and retrieve numbers from string for typecasting.